guid validator
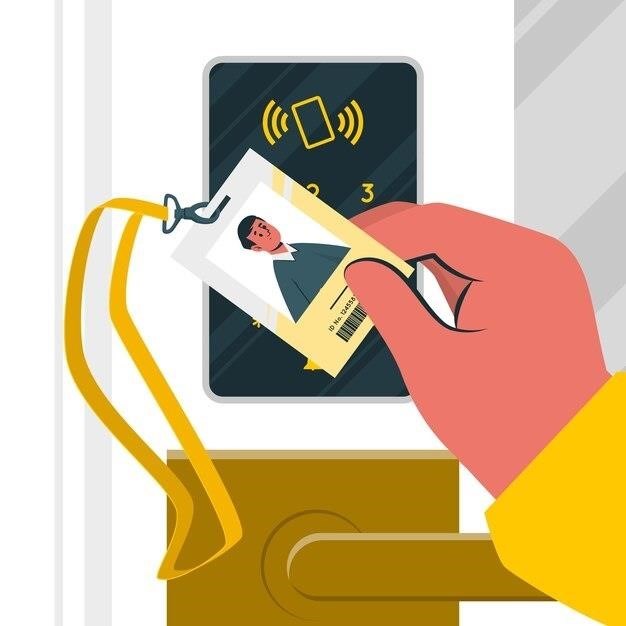
What is a GUID?
A GUID, which stands for Globally Unique Identifier, is a 128-bit integer number used to uniquely identify resources. It is also known as a UUID (Universally Unique Identifier). GUIDs are widely used in software development, particularly with Microsoft technologies, while UUIDs are more commonly used elsewhere.
Globally Unique Identifier
The term “Globally Unique Identifier” emphasizes the core purpose of a GUID⁚ to provide a unique identifier that is distinct across all systems and applications. It guarantees that no two GUIDs will ever be the same, even if generated simultaneously on different computers. This makes GUIDs ideal for situations where a universally recognized and unambiguous identifier is crucial, such as database records, file systems, and distributed applications.
Universally Unique Identifier
The term “Universally Unique Identifier” underscores the global scope of GUIDs. This means that a GUID generated on one computer will be recognized and understood by any other computer in the world, regardless of its operating system, network, or geographical location. This universal recognition is a key feature that enables seamless communication and data exchange across diverse systems.
Purpose
GUIDs serve as unique identifiers, ensuring that each resource or object has a distinct label. This is crucial for preventing conflicts and ensuring proper identification, especially when working with large datasets, distributed systems, or databases. The purpose of a GUID validator is to confirm that a given string adheres to the established format and structure of a GUID, thereby ensuring its validity and preventing potential errors or inconsistencies.
GUID Structure
A GUID is a 128-bit value, represented as a 36-character string, with five groups separated by hyphens. Each group consists of hexadecimal digits, ensuring a unique identifier.
128-bit Integer
At its core, a GUID is a 128-bit integer. This means it’s a number that uses 128 binary digits (bits) to represent its value. This large number of bits ensures a vast potential for unique identifiers, making collisions extremely unlikely. The 128-bit structure allows for a nearly infinite number of possible GUIDs, which is crucial for generating truly unique identifiers across various systems and applications.
While a GUID is technically a 128-bit integer, it’s typically represented as a 36-character string. This string consists of 32 hexadecimal digits, each representing four bits of the GUID’s value. These digits are grouped into five sections separated by hyphens, resulting in a familiar format like “89798912-1213-4545-9898-989898989898.” This string representation makes GUIDs easier to read and manage, while still maintaining the unique identifier’s integrity.
Hexadecimal Digits
The within a GUID string are hexadecimal digits, ranging from 0 to 9 and A to F. Each hexadecimal digit represents a group of four bits within the 128-bit GUID. The hexadecimal system uses base 16, allowing it to represent numbers more compactly than the decimal system. This compact representation is crucial for storing and transmitting GUIDs efficiently, especially when dealing with large datasets or network traffic.
Hyphens
Hyphens are crucial in the structure of a GUID, serving as separators between groups of hexadecimal digits. They divide the 32-character hexadecimal string into five distinct groups, making it easier to read and interpret. The hyphens also play a role in ensuring that the GUID remains a valid identifier, as they maintain the specific format required for identification and validation purposes. Without these hyphens, the GUID might be mistaken for a simple hexadecimal string, leading to potential errors or inconsistencies.
GUID Validation
Validating GUIDs is essential for ensuring data integrity and the proper functioning of systems that rely on unique identification.
Regular Expressions
Regular expressions offer a powerful and efficient method for validating GUIDs. These expressions define patterns that a string must match to be considered a valid GUID. A common pattern used in JavaScript for GUID validation is⁚ /^[0-9a-fA-F]{8}-[0-9a-fA-F]{4}-[0-9a-fA-F]{4}-[0-9a-fA-F]{4}-[0-9a-fA-F]{12}$/
. This pattern ensures the presence of 32 hexadecimal characters separated by four hyphens in the correct order.
Built-in Validators
Many programming languages and frameworks provide built-in functions for validating GUIDs. For instance, in C#, you can use the Guid.TryParse
method. This method attempts to parse a string into a GUID and returns a boolean value indicating whether the operation was successful. If the parsing is successful, the method also populates a Guid
object with the parsed value. This approach offers a convenient and reliable way to validate GUIDs within your code.
.NET 4.0
In .NET 4.0, the Guid.Parse
and Guid.TryParse
methods provide efficient ways to validate GUIDs. The Guid.Parse
method attempts to parse a string into a GUID, throwing a FormatException
if the string is invalid. On the other hand, the Guid.TryParse
method provides a safer alternative by returning a boolean value indicating success or failure without throwing an exception. This makes it suitable for scenarios where you need to handle invalid input gracefully.
GUID Applications
GUIDs play a crucial role in ensuring data integrity and verifying unique identifiers across various applications.
Unique Identifier Verification
GUIDs are essential for guaranteeing the uniqueness of identifiers in databases, ensuring that each record has a distinct and unambiguous reference. During database transactions and data exchange, GUID validation ensures the accuracy and consistency of identifiers, preventing data duplication and conflicts. This is crucial for maintaining data integrity and reliability across systems.
Data Integrity
Maintaining data integrity is paramount in various aspects of software development and system administration. GUID validation ensures that data stored in system logs, configuration files, and APIs adhere to the standardized structure of GUIDs, preventing inconsistencies and errors that could lead to system malfunctions or data corruption. This adherence to the standard format promotes reliable data management and ensures that data is consistent and trustworthy.
Online GUID Validators
Several online tools are available to validate GUIDs, including IsGUID.com, Aktos, and Free Online GUID Validator, providing quick and convenient verification of GUID format and structure.
IsGUID.com
IsGUID.com is a dedicated online tool for validating UUIDs and GUIDs. It analyzes the input string, checking its length, characters, and format against the UUID/GUID standards. The website offers a straightforward interface where you can enter your GUID and click “Validate” to receive instant feedback on whether it’s a valid identifier. This tool is particularly useful for developers and system administrators who need to ensure the integrity of GUIDs used in their applications and databases.
Aktos
Aktos offers a free online tool that provides guidance on validating GUIDs using JavaScript and regular expressions. The platform demonstrates the regex pattern used for validation, along with a JavaScript function illustrating its implementation. Aktos emphasizes the significance of GUID validation for web applications, highlighting its role in ensuring data integrity and security. The resource aims to equip developers with the necessary tools and knowledge to implement robust GUID validation within their web projects.
Free Online GUID Validator
This online tool allows users to quickly verify if a given string is a valid GUID. It simplifies the process by eliminating the need for manual character counting or format analysis. Users can simply input the string and click the “Test and Validate” button to receive immediate feedback. This resource is a valuable tool for developers and anyone working with GUIDs, providing a convenient and reliable way to ensure the accuracy of their identifiers.
GUID Generation
GUIDs are typically generated using algorithms that ensure their uniqueness. Microsoft technologies provide built-in functions for generating GUIDs, while other platforms utilize UUID generation methods.
Microsoft Technologies
Within Microsoft’s development ecosystem, the System;Guid
class provides functionality for generating GUIDs. The NewGuid
method within this class generates a new GUID based on a combination of the system’s current time, process ID, and other unique identifiers. This approach helps ensure that the generated GUIDs are highly unlikely to collide with each other, even across different systems or applications.
UUID Generation
The generation of UUIDs (Universally Unique Identifiers) typically involves a combination of random numbers, system-specific data, and timestamps. Various libraries and tools are available for generating UUIDs across different programming languages. Popular libraries include the uuid
library in Python and the java.util.UUID
class in Java. These libraries offer different algorithms and methods for generating UUIDs, ensuring their uniqueness and randomness.
Understanding GUIDs
To fully understand GUIDs, it’s important to grasp their versions, formats, and how they are encoded for use in Uniform Resource Identifiers (URIs).
Versions
GUIDs are defined by various versions, each with its own specific structure and generation method. Version 1 GUIDs, for instance, are based on timestamps and MAC addresses, making them suitable for tracking changes over time. Version 4 GUIDs, on the other hand, utilize random numbers, ensuring uniqueness even without access to network resources. Understanding the version helps in interpreting the structure and purpose of a particular GUID;
Formats
GUIDs are typically represented as 36-character hexadecimal strings separated by hyphens. This format is commonly used for readability and easy parsing. However, GUIDs can also be represented in other formats, such as binary, decimal, or URI-encoded strings. These alternative formats might be used in specific contexts, such as data transmission or storage. A GUID validator should be able to handle various formats to ensure accurate verification.
URI Encoding
In certain scenarios, GUIDs might need to be encoded for use in Uniform Resource Identifiers (URIs). This is because some characters in GUIDs, such as hyphens, are reserved characters in URI specifications. URI encoding replaces these characters with their corresponding percent-encoded equivalents. A GUID validator should be able to handle URI-encoded GUIDs and decode them correctly to perform validation.
Validating GUIDs is crucial for ensuring data integrity and consistency across systems. Online tools and libraries provide convenient methods for verifying the format and structure of GUIDs, safeguarding data integrity and ensuring reliable system operations.
Importance of GUID Validation
Validating GUIDs is essential for maintaining data integrity and consistency across systems. Incorrectly formatted GUIDs can lead to errors in database transactions, data exchange, and system logs. By ensuring that GUIDs adhere to the standard format, developers can prevent these issues and maintain the reliability of their applications. Furthermore, validation plays a crucial role in protecting against potential security vulnerabilities, as invalid GUIDs could be exploited for malicious purposes.
Tools and Resources
Numerous online tools and resources are available to assist developers in validating GUIDs. Websites like IsGUID.com, Aktos, and Free Online GUID Validator provide user-friendly interfaces for checking the format and structure of GUIDs. These platforms offer a convenient way to quickly verify the validity of GUIDs without requiring complex code implementations. Additionally, programming languages like C# and JavaScript provide built-in functions for validating GUIDs, enabling developers to integrate this functionality directly into their applications.