effective c pdf
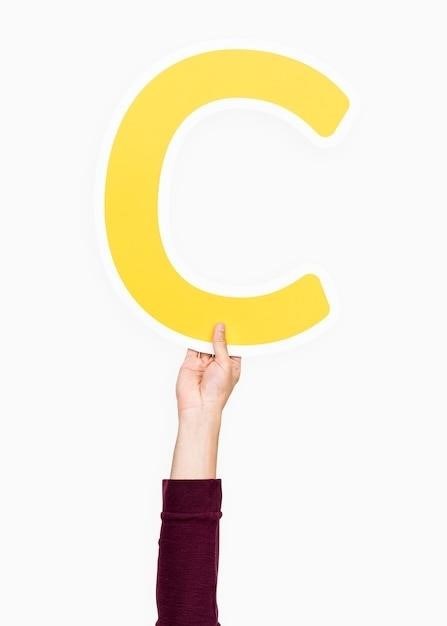
Effective C Programming⁚ A Comprehensive Guide
This guide delves into the intricacies of effective C programming, covering fundamental concepts, advanced techniques, and real-world applications. It’s a comprehensive resource for programmers of all levels, from beginners to seasoned professionals. Whether you’re looking to enhance your C skills or embark on a journey to master this powerful language, this guide provides a structured path to achieve your goals.
Introduction to Effective C
The C programming language, renowned for its efficiency and versatility, has long been a cornerstone of software development. Its power lies in its ability to interact directly with hardware, enabling developers to create high-performance applications. However, harnessing the full potential of C requires a deep understanding of its nuances and best practices. This comprehensive guide, “Effective C⁚ An Introduction to Professional C Programming,” serves as your roadmap to mastering this powerful language.
This guide goes beyond the basics, delving into the intricacies of effective C programming. It guides you through the fundamental concepts, advanced techniques, and real-world applications that empower you to write robust and efficient code. Whether you are a seasoned programmer seeking to refine your C skills or a novice embarking on your coding journey, this guide provides a structured path to proficiency.
This guide is not merely a theoretical treatise; it is a practical guide to professional C programming, offering insights gleaned from real-world experiences. It explores the challenges developers encounter in the real world and provides solutions based on proven techniques and best practices. You’ll learn how to avoid common pitfalls, optimize performance, and write code that is both efficient and maintainable.
Throughout this guide, you will find clear explanations, practical examples, and insightful advice that will help you develop a deep understanding of C. It is a valuable resource for anyone seeking to enhance their C programming skills and embark on a journey towards becoming a proficient C developer.
Understanding C Fundamentals
The foundation of effective C programming lies in a solid grasp of its fundamental concepts. This section delves into the core elements of the C language, providing a comprehensive understanding of its syntax, data types, operators, and control flow. Understanding these fundamentals is crucial for writing clear, concise, and efficient C code.
Data Types⁚ C supports a variety of data types, each designed to represent specific kinds of data. You’ll learn about integers, floating-point numbers, characters, and the role of data type declarations in defining variables. Understanding the appropriate data types for different tasks is essential for writing efficient and accurate code.
Operators⁚ Operators are the symbols that perform operations on data. This section explores arithmetic operators (+, -, *, /, %), relational operators (>, <, >=, <=, ==, !=), logical operators (&&, ||, !), and bitwise operators (&, |, ^, ~, <<, >>). Understanding these operators is critical for manipulating data and controlling program flow.
Control Flow⁚ Control flow statements dictate the order in which instructions are executed. You’ll learn about if-else statements, for loops, while loops, and switch statements. These statements allow you to create programs that make decisions, repeat actions, and handle various scenarios.
Functions⁚ Functions are blocks of code that perform specific tasks. You’ll learn about function definition, function calls, parameter passing, and return values. Functions are essential for organizing code, promoting reusability, and creating modular programs.
This section provides a solid foundation in C fundamentals, empowering you to write basic C programs. Building upon this understanding, we will explore more advanced concepts and techniques in the following sections.
Advanced C Concepts
This section dives deeper into the world of C programming, exploring concepts that go beyond the fundamentals. Mastering these advanced techniques will unlock a greater level of control and efficiency in your C code, allowing you to tackle complex programming challenges.
Pointers⁚ Pointers are variables that store memory addresses. They are a powerful tool for accessing and manipulating data directly in memory. You’ll learn about pointer declaration, pointer arithmetic, and the use of pointers to access array elements and dynamically allocate memory.
Arrays⁚ Arrays are collections of elements of the same data type stored contiguously in memory. You’ll learn about array declaration, array indexing, multi-dimensional arrays, and passing arrays to functions. Arrays are fundamental for storing and processing large datasets.
Structures⁚ Structures allow you to group related data elements together under a single name. You’ll learn about structure declaration, member access, and passing structures to functions. Structures provide a way to create custom data types that represent real-world entities.
Memory Management⁚ C provides mechanisms for allocating and managing memory dynamically. You’ll learn about dynamic memory allocation using malloc, calloc, and realloc, and the importance of freeing allocated memory using free. Efficient memory management is crucial for preventing memory leaks and ensuring program stability.
File I/O⁚ C provides functions for interacting with files. You’ll learn about file opening, file reading, file writing, and file closing. File I/O is essential for storing and retrieving data persistently.
This section introduces you to advanced C concepts that enhance your programming abilities and enable you to build more sophisticated and efficient programs. By mastering these concepts, you can leverage the full power of the C language.
Memory Management in C
Memory management is a crucial aspect of C programming, as it directly impacts the efficiency and stability of your code. C gives you a lot of control over how memory is used, but this freedom also comes with responsibility. Understanding and practicing effective memory management techniques is essential to avoid common pitfalls like memory leaks and crashes.
Dynamic Memory Allocation⁚ Unlike statically declared variables that have fixed memory locations, C allows you to allocate memory dynamically during program execution. This is achieved through the use of functions like `malloc`, `calloc`, and `realloc`. `malloc` allocates a block of memory of a specified size, `calloc` allocates a block of memory and initializes it to zero, and `realloc` allows you to resize an already allocated memory block.
Freeing Memory⁚ Dynamically allocated memory must be explicitly freed when it’s no longer needed. This is done using the `free` function. Failing to free allocated memory results in memory leaks, where the program consumes more and more memory over time, potentially leading to crashes or performance issues.
Memory Leaks⁚ A memory leak occurs when dynamically allocated memory is no longer referenced by the program but remains occupied. This can happen if a pointer to the allocated memory is lost or overwritten, or if a program terminates without freeing all allocated memory.
Pointers and Memory Management⁚ Pointers are intricately linked to memory management in C. They provide direct access to memory locations. When you use pointers for dynamic memory allocation, it’s crucial to handle them carefully to avoid accessing invalid memory locations.
Importance of Memory Management⁚ Effective memory management is essential for⁚
- Preventing memory leaks and improving program stability.
- Optimizing program performance by managing memory efficiently.
- Ensuring that your program doesn’t consume more memory than it needs.
This section provides a foundational understanding of memory management in C, emphasizing the importance of proper allocation, freeing, and handling of memory. Mastering these concepts is crucial for writing robust and efficient C programs.
Pointers and Arrays in C
Pointers and arrays are fundamental concepts in C that are closely intertwined and play a critical role in memory management, data manipulation, and algorithm optimization. Understanding their relationship and effective usage is essential for writing efficient and robust C programs.
Pointers⁚ A pointer is a variable that stores the memory address of another variable. They are declared using the `*` operator, and their values can be assigned using the `&` operator, which returns the memory address of a variable. Pointers provide a powerful mechanism for direct memory access and manipulation.
Arrays⁚ An array is a collection of elements of the same data type stored in contiguous memory locations. Array elements are accessed using an index, starting from 0. Arrays are often used to store collections of data, such as lists of numbers, characters, or structs.
Relationship between Pointers and Arrays⁚ In C, the name of an array decays into a pointer to its first element. This means that you can use a pointer to access and modify the elements of an array. For example, if `arr` is an array of integers, `arr` can be used as a pointer to the first element, and `arr + i` points to the i-th element.
Advantages of Using Pointers with Arrays⁚
- Dynamic Memory Allocation⁚ Pointers can be used to dynamically allocate memory for arrays, allowing you to create arrays of variable sizes during program execution.
- Efficient Data Access⁚ Pointers provide direct access to memory locations, enabling faster data manipulation compared to array indexing.
- Function Parameters⁚ Pointers can be passed to functions as arguments, allowing functions to modify the original array data.
- String Manipulation⁚ Pointers are extensively used in string manipulation, enabling operations like character-by-character processing, string concatenation, and substring extraction.
This section highlights the close relationship between pointers and arrays in C. Understanding their interplay and effective usage is vital for writing efficient and dynamic C programs.
Data Structures in C
Data structures are fundamental building blocks in programming that organize and store data in a way that facilitates efficient access and manipulation. C provides a powerful set of data structures, allowing programmers to create sophisticated and flexible data representations tailored to specific applications. Understanding and effectively utilizing these structures are essential for building efficient, organized, and maintainable C programs.
Fundamental Data Structures in C⁚
- Arrays⁚ Arrays are collections of elements of the same data type stored in contiguous memory locations. They are ideal for storing lists of data, such as a list of student names or a series of temperature readings.
- Structures⁚ Structures (structs) allow you to group variables of different data types under a single name. This facilitates representing complex data, such as a student record containing name, age, and grade.
- Unions⁚ Unions are similar to structs but allow different members to share the same memory location. This can be used to save memory when only one member is needed at a time.
- Enums⁚ Enums (enumerations) define a set of named integer constants. They improve code readability and maintainability by providing meaningful names for constants.
Advanced Data Structures in C⁚
In addition to these fundamental structures, more advanced data structures can be implemented in C using pointers and dynamic memory allocation⁚
- Linked Lists⁚ Linked lists are dynamic data structures where elements are connected through pointers. Each element, or node, contains data and a pointer to the next node in the list. Linked lists provide flexibility for inserting and deleting elements without requiring contiguous memory.
- Stacks⁚ Stacks are data structures that follow the Last-In, First-Out (LIFO) principle. Elements are added (pushed) and removed (popped) from the top of the stack. Stacks are often used for function call management and expression evaluation.
- Queues⁚ Queues are data structures that follow the First-In, First-Out (FIFO) principle. Elements are added (enqueued) at the rear and removed (dequeued) from the front. Queues are used in various applications, such as job scheduling and message handling.
- Trees⁚ Trees are hierarchical data structures where elements are organized in a parent-child relationship; They are often used for efficient searching and sorting operations. Common types of trees include binary trees, AVL trees, and B-trees.
- Graphs⁚ Graphs are data structures that represent relationships between objects (nodes) using edges. They are used in various applications, such as social networks, transportation networks, and web page analysis.
Mastering data structures in C is crucial for efficient data management, algorithm design, and problem-solving in a wide range of applications. Understanding the fundamental structures, their implementation, and their strengths and weaknesses will empower you to choose the right structure for your specific needs.
File Handling in C
File handling is an essential aspect of C programming, allowing programs to interact with files stored on the computer’s storage system. This interaction enables programs to read data from files, write data to files, and manage file operations. Effective file handling is crucial for applications that require persistent data storage, such as database management systems, text editors, and configuration files.
C provides a set of standard library functions that facilitate file handling operations. These functions allow programmers to open, read, write, close, and manipulate files, providing a structured and reliable way to interact with the file system.
Key File Handling Functions⁚
- fopen⁚ Opens a file, establishing a connection between the program and the file. It takes the file path and mode (read, write, append) as arguments.
- fclose⁚ Closes a file, releasing resources and ensuring data integrity.
- fread⁚ Reads data from an open file into a buffer. It takes the buffer, size of data to read, number of items to read, and file pointer as arguments.
- fwrite⁚ Writes data from a buffer to an open file. It takes the buffer, size of data to write, number of items to write, and file pointer as arguments.
- fgetc⁚ Reads a single character from an open file.
- fputc⁚ Writes a single character to an open file.
- fgets⁚ Reads a line of text from an open file.
- fputs⁚ Writes a string of text to an open file.
- fseek⁚ Moves the file pointer to a specific position within a file. This allows for random access to data within the file.
- ftell⁚ Returns the current position of the file pointer within a file.
- rewind⁚ Moves the file pointer to the beginning of the file.
File Modes⁚
Different file modes are used to specify the type of access required for a file⁚
- “r”⁚ Open for reading (default mode).
- “w”⁚ Open for writing (creates a new file or overwrites an existing file).
- “a”⁚ Open for appending (appends data to the end of an existing file or creates a new file if it doesn’t exist).
- “r+”⁚ Open for reading and writing.
- “w+”⁚ Open for reading and writing (creates a new file or overwrites an existing file).
- “a+”⁚ Open for reading and appending (appends data to the end of an existing file or creates a new file if it doesn’t exist).
By understanding the various file handling functions and modes, programmers can effectively manage and manipulate files, enabling their C programs to interact with data stored on the computer’s file system.
Error Handling and Debugging
Error handling and debugging are critical aspects of effective C programming. These practices ensure that programs run smoothly, handle unexpected situations gracefully, and provide developers with the tools to identify and resolve issues efficiently; Effective error handling and debugging practices are crucial for creating robust and reliable C applications.
Error Handling⁚
Error handling in C involves anticipating potential problems that might arise during program execution and implementing mechanisms to handle these situations gracefully. This includes⁚
- Input Validation⁚ Checking user inputs to ensure they conform to expected formats and ranges, preventing invalid data from causing errors.
- Resource Management⁚ Properly allocating and releasing resources (memory, files, network connections) to avoid leaks and other resource-related issues.
- Exception Handling⁚ Using mechanisms (like `try…catch` blocks in C++) to handle exceptional conditions that may disrupt program flow.
- Error Logging⁚ Recording error messages and details to facilitate debugging and analysis of problems.
Debugging Techniques⁚
Debugging involves identifying and resolving errors in C programs. Common debugging techniques include⁚
- Print Statements⁚ Inserting `printf` statements to display variable values and program flow, helping to track down the source of errors.
- Debuggers⁚ Using specialized tools (like GDB) to step through program execution, inspect variables, and identify the root cause of issues.
- Code Review⁚ Having other developers examine the code for potential errors and inconsistencies.
- Unit Testing⁚ Writing tests to verify the functionality of individual code modules, catching errors early in the development process.
Error Handling Best Practices⁚
- Use Assertions⁚ Include `assert` statements to enforce conditions and catch errors early in development.
- Handle Errors Gracefully⁚ Provide informative error messages to users and log errors for debugging.
- Test Thoroughly⁚ Execute the program with various inputs and scenarios to identify potential errors.
- Document Error Handling⁚ Clearly document error handling strategies and potential error conditions.
By adopting effective error handling and debugging practices, developers can create C programs that are robust, reliable, and easier to maintain, leading to a more efficient and productive development process.